일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | |||
5 | 6 | 7 | 8 | 9 | 10 | 11 |
12 | 13 | 14 | 15 | 16 | 17 | 18 |
19 | 20 | 21 | 22 | 23 | 24 | 25 |
26 | 27 | 28 | 29 | 30 | 31 |
- Dijkstra
- 다익스트라
- 참고 문헌 : MACHINE LEARNING 기계학습 _ 오일석
- 다이나믹프로그래밍
- MySQL
- 최대공약수
- sql고득점kit
- 스택
- 시스템콜
- 백준
- String
- 리시프
- unordered_map
- 알고리즘
- 문자열
- 코테준비
- 코딩테스트연습
- substr
- 동적계획법
- 우선순위큐
- DP
- C++
- vector
- 플로이드와샬
- 코테
- 프로그래머스
- dfs
- MAP
- 정렬
- set
- Today
- Total
YeJin's Footsteps
OpenGL 응용1 본문
Model Transformation
void glLoadIdentity(); // 항등 행렬로 초기화
void glMatrixMode(GLenum mode)
- GL_MODELVIEW // 제일 많이 씀(물체를 움직일 때)
- GL_PROJECTION // 얼마만큼의 3차원을 어떤방식의 2차원으로 보내줄 지
- GL_TEXTURE // 물체 표면의 질감
void glRotatef(GLfloat angle, GLfloat x, GLfloat y, GLfloat z) // 회전 함수
void glTranslatef(GLfloat dx, GLfloat dy, GLfloat dz) //이동 함수
void glScalef(GLfloat sx, GLfloat sy, GLfloat sz) //크기 조절함수 (1.0, 1.0, 1.0)이 original scale
glRotatef, glTranslatef Example
#include <stdlib.h>
#include <glut.h>
#include <GL/GL.h>
#include <GL/GLU.h>
void MyDisplay(){
glClear(GL_COLOR_BUFFER_BIT); // GL상태변수 설정, 프레임 버퍼를 초기화 // 초기화 될 색은 glutClearColor에서 사용된 색
glViewport(0, 0, 300, 300); //화면을 다 쓰겠다.
glMatrixMode(GL_MODELVIEW);
//하늘색 사각형
glColor3f(0.0, 0.9, 0.9);
glLoadIdentity();
glutSolidCube(0.3);
//분홍색 사각형
glColor3f(0.9, 0.0, 0.9);
glLoadIdentity();
glRotatef(45.0, 0.0, 0.0, 1.0);
glTranslatef(0.6, 0.0, 0.0);
glutSolidCube(0.3);
//노란색 사각형
glColor3f(0.9, 0.9, 0.0);
glLoadIdentity();
glTranslatef(0.6, 0.0, 0.0);
glRotatef(45.0, 0.0, 0.0, 0.1);
glutWireCube(0.3);
glFlush();
}
int main(int argc, char** argv){
glutInit(&argc, argv); // GLUT 윈도우 함수
glutInitDisplayMode(GLUT_RGB); // 윈도우의 기본컬러모드를 RGB모드로 설정
glutInitWindowSize(300, 300); // 윈도우 사이즈 설정
glutCreateWindow("OpenGL example");
glClearColor(1.0, 1.0, 1.0, 1.0); // GL 상태변수 설정, 마지막 알파값은 1이면 불투명 0이면 투명
glMatrixMode(GL_PROJECTION); //
glLoadIdentity();
glOrtho(-1.0, 1.0, -1.0, 1.0, -1.0, 1.0);
glutDisplayFunc(MyDisplay); // GLUT 콜백함수 등록
glutMainLoop(); // 이벤트 루프 진입
return 0;
}
회전, 이동의 순서에 따라 결과가 달라짐
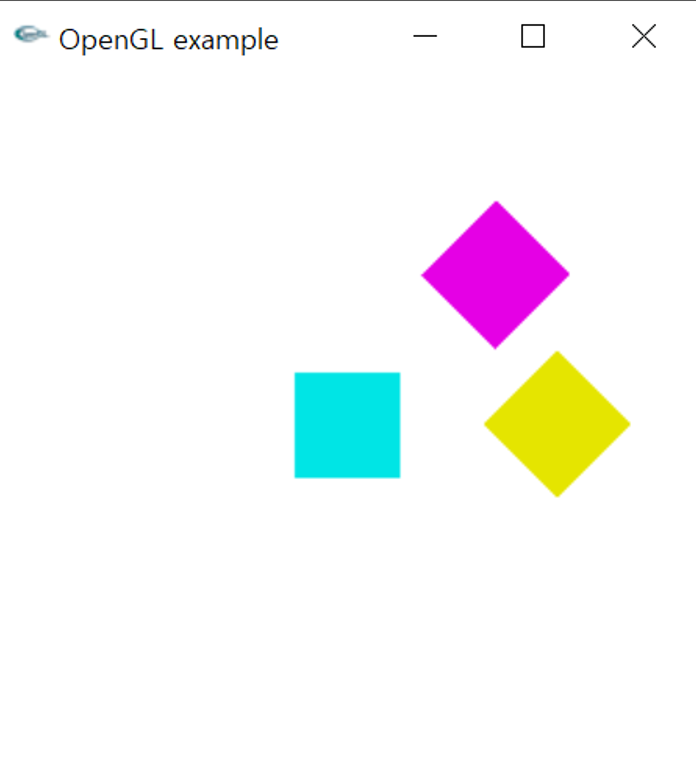
void gluLookAt(GLdouble eyex, GLdouble eyey, GLdouble eyez, GLdouble atx, GLdouble aty, GLdouble atz, GLdouble upx, GLdouble upy, GLdouble upz) *upx, upy, upz : Camera Up-vector
default : gluLookAt(0.0, 0.0, 0.0, 0.0, 0.0, -100.0, 0.0, 1.0, 0.0)
gluLookAt Example
#include <stdlib.h>
#include <glut.h>
#include <GL/GL.h>
#include <GL/GLU.h>
void MyDisplay(){
glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); // GL상태변수 설정, 프레임 버퍼를 초기화 // 초기화 될 색은 glutClearColor에서 사용된 색
glViewport(0, 0, 400, 400); //화면을 다 쓰겠다.
glMatrixMode(GL_MODELVIEW);
glEnable(GL_DEPTH_TEST);
glLoadIdentity();
glColor3f(0.3, 0.3, 0.3);
gluLookAt(0.0, 0.0, 0.0, 0.0, 0.0, -1.0, 1.0, 1.0, 0.0);
glutWireTeapot(0.5);
glFlush();
}
int main(int argc, char** argv){
glutInit(&argc, argv); // GLUT 윈도우 함수
glutInitDisplayMode(GLUT_RGB|GLUT_RGBA | GLUT_DEPTH); // 윈도우의 기본컬러모드를 RGB모드로 설정
glutInitWindowSize(400, 400); // 윈도우 사이즈 설정
glutCreateWindow("OpenGL example");
glClearColor(1.0, 1.0, 1.0, 1.0); // GL 상태변수 설정, 마지막 알파값은 1이면 불투명 0이면 투명
glutDisplayFunc(MyDisplay); // GLUT 콜백함수 등록
glutMainLoop(); // 이벤트 루프 진입
return 0;
}
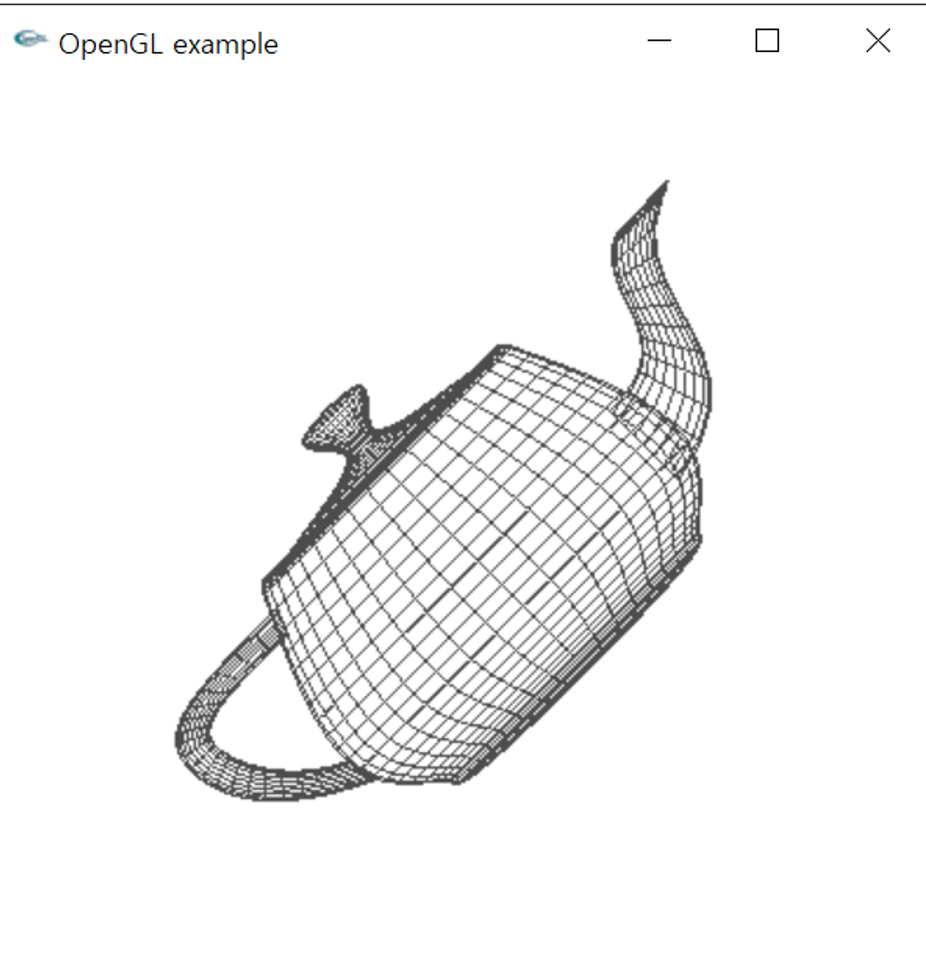
void glOrtho //멀리있던 가까이 있던 똑같은 크기
void glFrustum , gluPerspective //사람이 보는 방식
#include <glut.h>
void display(void) {
glClear(GL_COLOR_BUFFER_BIT);
glShadeModel(GL_FLAT);
glColor3f(0.0, 0.0, 1.0);
glLoadIdentity();
gluLookAt(1.0, 0.0, 10.0, 0.0, 0.0, 0.0, 0.0, 1.0, 0.0);
glScalef(1.0, 2.0, 1.0);
glutWireTeapot(3.0);
glFlush();
}
void reshape(int w, int h) {
glViewport(0, 0, (GLsizei)w, (GLsizei)h);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
glFrustum(-1.0, 1.0, -1.0, 1.0, 1.5, 20.0);
glMatrixMode(GL_MODELVIEW);
}
int main(int argc, char** argv) {
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_SINGLE | GLUT_RGB);
glutInitWindowSize(800, 400);
glutCreateWindow("OpenGL Sample Drawing");
glClearColor(1.0, 1.0, 1.0, 1.0);
glutDisplayFunc(display);
glutReshapeFunc(reshape);
glutMainLoop();
return 0;
}
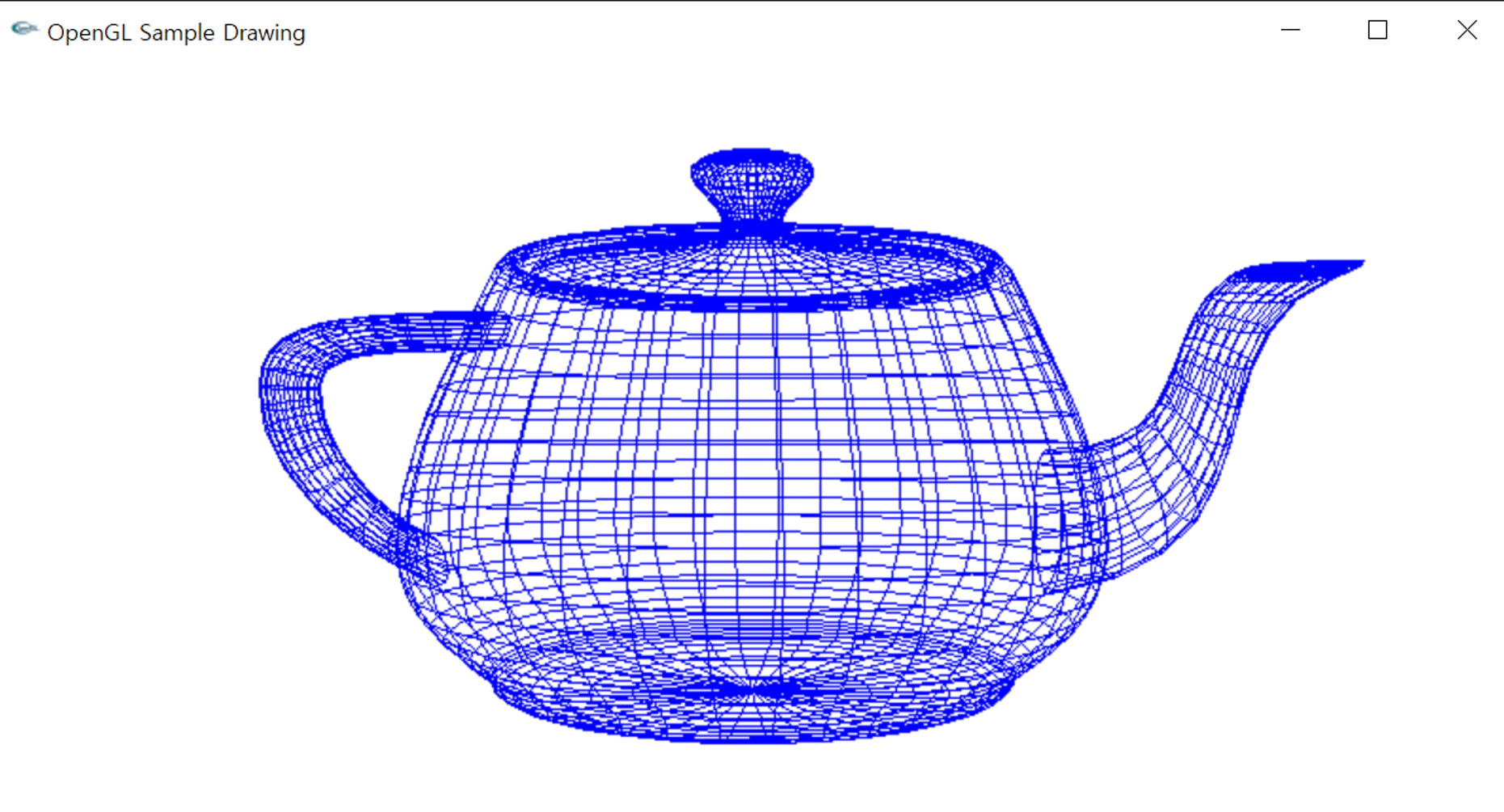
'Computer Science & Engineering > OpenGL' 카테고리의 다른 글
OpenGL 기초 (실습) (0) | 2021.04.05 |
---|